Win 10 x64 10.0.17134.590
Apache 2.24.33 x64 vc15 version
Php 7.2.11 x64 vc15 version
MySQL 8.0.11 x86_64 version port 3306
mysql-connector-net-6.10.8.msi
mysql-for-visualstudio-2.0.5.msi
phpMyAdmin 4.85
remember a few things
1. you need to use a simple setup like a router at 192.168.1.1
2. mysql server for example at a static 192.168.1.2 (see network connections to make it static)
3. mysql clients at 192.168.1.3 and 4,5,6,7,... etc
4. mysql by default doesn't allow anything but localhost (127.0.0.1) or here (192.168.1.2) to access it
5a. you can change that with these commands (using user name ip and password commands to mysql - i use php to do it)
5b. if you want, just create users by hand in a mysql command prompt or the mysql workbench app for windows, etc.
https://stackoverflow.com/questions/623 ... r-for-user
PHP Style
setup an admin maybe [admin][abcdefgh!]
structure of web in htdocs is as such
[site]
|_____[mS]
.....|_____[admin] info.php
.....|_____[dancers] init_clients.php
_info.php example
Code: Select all
<?php
//when you include or require a file that has code, it has to have the php code beg and end parts or it will basically echo as text
$companyTitle="risque";
$company="risque";
$db=$company;
//
//localhost
$admin="admin";
$adminPass="abcdefgh!";
$server="localhost";
$user="johnDoe";
$pass="12345678!";
?>
Code: Select all
<?php
/*
02-28-2019
init_clients.php v1.0 by kristoffe brodeur. ©2019 All Rights Reserved.
requires init_db.php to run first so local database is setup
also mysql variables are in admin area of _info.php
*/
$to_root="../../";
require $to_root."mS/admin/_info.php";
/*
setup ip addresses 3,4,5,6 as in 192.168.1.3 192.168.1.4 192.168.1.5...
*/
$ipArr=explode(",","3,4,5,6");
$ipLen=count($ipArr);
//
echo '<h5>Create ['.$ipLen.'] new user access rights for <span class="ok">'.$db.'</span></h5><hr />';
$connect=mysqli_connect($server,$user,$pass,$db);
/*
$queryStr="CREATE USER 'johnDoe'@'192.168.1.5' IDENTIFIED BY 12345678!';";
$queryStr="GRANT ALL ON dbExample.* TO 'johnDoe'@'192.168.1.5'";
added mQuery to make multiple adds and grants robust, verbose and simple
*/
//
function mQuery($qStr)
{
echo "[query]$qStr<br />";
mysqli_query($connect,$qStr);
$err=mysqli_error($connect);
//
if($err=="")
{
echo "sucessful.<br />";
}
else
{
echo "<br />[error]$err<br />";
}
}
//
for($a=0;$a<$ipLen;$a++)
{
$ipStr="192.168.1.".$ipArr[$a];
mQuery("CREATE USER '$admin'@'$ipStr' IDENTIFIED BY '$adminPass';");
mQuery("GRANT ALL ON dbExample.* TO '$admin'@'$ipStr'");
mQuery("CREATE USER '$user'@'$ipStr' IDENTIFIED BY '$pass';");
mQuery("GRANT ALL ON dbExample.* TO '$user'@'$ipStr'");
}
mysqli_close($connect);
echo "<hr />Connection closed.<br />";
?>
Add Mysql.Data reference to your Form, Form1.cs for example
solution explorer -> References -> right click Add Reference
Assemblies -> Extensions -> MySql.Data 8.0.15.0, check mark (OK)
at the top, in the using area, add
Code: Select all
using MySql.Data.MySqlClient;
you can make a list of users to have names and passwords in the database as well
Code: Select all
string ConnectionString = "server=127.0.0.1;Database=dbExample;Uid=johnDoe;Pwd=12345678!;";
MySqlConnection con = new MySqlConnection(ConnectionString);
This is partial and inside of a public form build, but still works with it being thread safe (DOT Net 4.x fix I band aided for now)
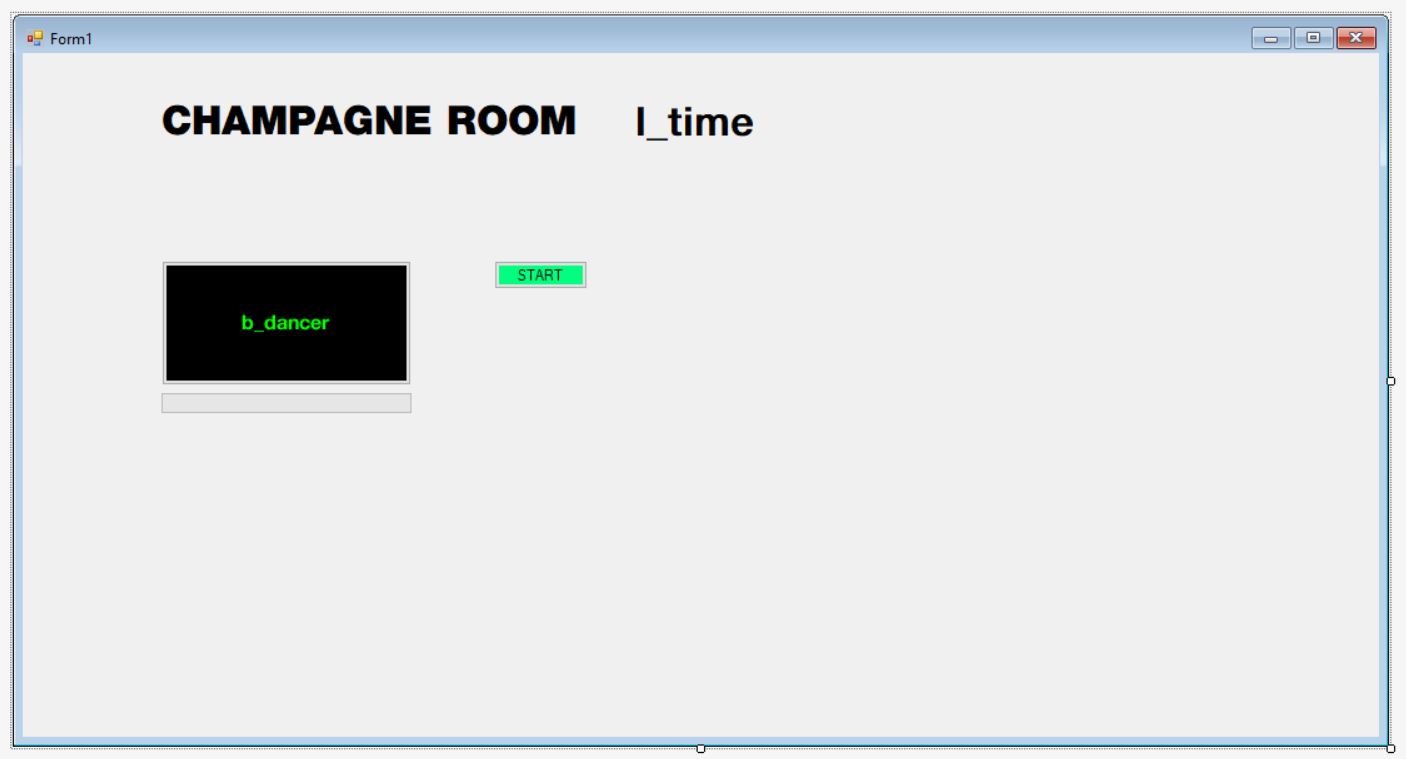
Code: Select all
using System;
using System.Timers;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
using System.Windows.Forms;
namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
public static System.Timers.Timer aTimer;
public void Form1_Load(object sender, EventArgs e)
{
string ConnectionString = "server=127.0.0.1;Database=dbExample;Uid=johnDoe;Pwd=12345678!;";
MySqlConnection con = new MySqlConnection(ConnectionString);
string QueryString = "SELECT * FROM dancers WHERE id='0001'";
MySqlCommand cmd = new MySqlCommand(QueryString, con);
con.Open();
MySqlDataReader reader = cmd.ExecuteReader();
string _dName = "";
//
while (reader.Read())
{
_dName = reader.GetString("_dName");
b_dancer.Text = _dName;
}
//
if (con.State == ConnectionState.Open)
{
con.Close();
}
aTimer = new System.Timers.Timer(1000);
aTimer.Elapsed += onTimedEvent;
aTimer.AutoReset = true;
aTimer.Enabled = true;
}
public void onTimedEvent(Object source, ElapsedEventArgs e)
{
string ConnectionString = "server=127.0.0.1;Database=dbExample;Uid=johnDoe;Pwd=12345678!;";
MySqlConnection con = new MySqlConnection(ConnectionString);
string QueryString = "SELECT NOW()";
MySqlCommand cmd = new MySqlCommand(QueryString, con);
con.Open();
MySqlDataReader reader = cmd.ExecuteReader();
string _serverTime = "";
//
while (reader.Read())
{
_serverTime = reader.GetString(0);
l_time.Invoke((MethodInvoker)(() => l_time.Text = _serverTime));
}
//
if (con.State == ConnectionState.Open)
{
con.Close();
}
}
}
}