It seems iPower has made it so by default just calling a session_start() will not save a session on their shared servers. This is bad for generic scripting and good overall for security and multiple session locations. One way to set a session location on iPower's shared servers is to make a folder [sessions] which is not hacker proof but if you want something safer you can declare it outside of the htdocs area. For now, just make a folder [sessions] in your root folder, and use the following code to reference where your session folder is for iPower's server setup:
just an example needed before each php program that calls sessions:
Code: Select all
$to_root="../../";
session_save_path($to_root."sessions");
session_start();
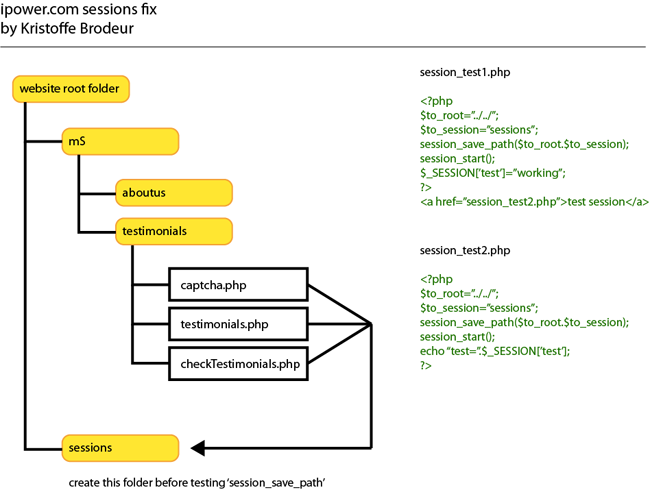

I wrote this to tutor on captcha but I wanted to make it more dynamic and stronger than just a simple inline program. We both explored the possibilities of our own simple captcha without image filters. Captcha in this way uses a crazy font I found online "KAIREE.TTF" which is not like any normal american a-z alphabet character pattern. This is great as it doesn't need to be blurry, twisted, etc, like most captchas seem to need to fool robots.
i also thought, what if you wanted to just store it as a stream without a filename, and for as many visitors the php engine can handle (apache's host is the big horse here)? so, by simply adding ?v=1 ?v=2 ?v=3 etc, inline
Code: Select all
<img src="captcha.php?v=*" />
don't forget to find a true type font that is free and you can save on your server. find 'KAIREE.TTF' or your own, and change the line that looks like this:
Code: Select all
$font="KAIREE.TTF";//must be in the folder you specify, save that ttf so php and gd2 can read it and use it to build characters
here is the code for captcha.php
Code: Select all
<?php
/*
captcha.php v1.0 by Kristoffe Brodeur ©2010 All Rights Reserved
10-29-2010
create a bitmap into php memory for the client that is not stored on the hard drive
so each call will be unique, no visitor will see the same image, multiple calls per browser can be made easily
until your server runs out of memory that is
http://www.php.net/manual/en/image.examples-png.php
*/
header("Content-type:image/png");//oh, I'm not html this time around, I'm letting the asking browser know I'm spitting out a png here
//-----almost exactly from dyn_img_text.php but NO SAVING to hard drive on server
/*
create a new image into a bitmap in memory only (in a var), not on the hard drive on the server
store a bitmap in a var not a string, pixels (80wx60h, etc) like a photoshop image
*/
$w=320;//pixels
$h=100;//pixels
/*
truecolor is 24 bits R(8)G(8)B(8) 255:0:0 (bright red)
32 bits is RGBA (alpha) a cool image with a transparent background, like a png round icon with wallpaper on a webpage
*/
$img=imageCreateTrueColor($w,$h);
//rgb 0-255 0=dark 255=total color or r,g, or b (like a dial for each) 66 dark red 255 chinese red
//same difference here
//$fC=new Array("66","89","104");
//$fCArr=explode(",","255,127,0");//take a string, and make an array with comma as the node seperator (explode it)
//$fontColor=imagecolorallocate($img,$fCArr[0],$fCArr[1],$fCarr[2]);
$fontColor=imagecolorallocate($img,255,127,0);
//same difference
//$fontColor=imagecolotallocate($img,$fCArr[0],$fCArr[1],$fCarr[2]);
/*
imagettftext
image bitmap
size of the text font
angle like italic in degrees? clockwise positive #(/) cc negative #(\)
x point to start text (left-right)
y point to start text (up-down)
font color in a imagecolorallocate with the bitmap and rgb in r,g,b (above)
font family and place the ttf font family is stored on the server so gd2 can read it to draw letters (KAIREE.TTF) file has to be where you say it is to reference and load into gd2 memory to draw
text to draw
*/
//$text="this should work!";
$len=8;
$chars="abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890";
$ranLen=strlen($chars);//how long is...
$text="";
//
for($a=0;$a<$len;$a++)
{
$pnt=rand(0,$ranLen);//random point to start
$ranChar=substr($chars,$pnt,1);//one char at that point
//echo $ranChar;
$text.=$ranChar;
}
//
$font="KAIREE.TTF";//must be in the folder you specify, save that ttf so php and gd2 can read it and use it to build characters
$fontSize=24;
$x=80;//move 80 to the right from the left edge
$y=32+$fontSize;
imagettftext($img,$fontSize,0,$x,$y,$fontColor,$font,$text);
$filename="test.png";//save the bitmap we dynamically create ($img) to the hard drive on the server ($filename) as test.png
//imagepng($img,$filename);
imagepng($img);//this time only a memory bitmap of the image, no value to a file save on the hard drive on the server
imagedestroy($img);//just clear up the server's memory from the scratch space used to temporarily create the image, like closing photoshop shen you've saved your doodle
?>
Code: Select all
<html>
<body>
<h3>Multiple Captcha calling a php image generator (press F5 to regenerate)</h3><hr />
<table>
<tr>
<td width="400px" valign="top">
<h4>This just shows that a php file can stream back a PNG and not just HTML</h4>
<p>in this example, it doesn't save anything to the had drive on the server or client (really) it just sends a temporary stream 'file' with an arbitrary name per browser per cache per image</p>
<p>also, the ?v=... means, pretend I am not asking for the same resource over and over. Try it with the same name or same v=1 on a few, it will repeat in those places. change a bogus GET var and you'll see unique images!</p>
</td>
<td>
<img src="captcha.php?v=1" /><br>captcha.php?v=1<br>
<img src="captcha.php?v=2" /><br>captcha.php?v=2<br>
<img src="captcha.php?v=3" /><br>captcha.php?v=3<br>
<img src="captcha.php?v=4" /><br>captcha.php?v=4<br>
<img src="captcha.php?v=5" /><br>captcha.php?v=5
</td>
</tr>
</table>
</body>
</html>
Code: Select all
<?php
/*
create a new image into a bitmap in memory only (in a var), not on the hard drive on the server
store a bitmap in a var not a string, pixels (80wx60h, etc) like a photoshop image
*/
$w=320;//pixels
$h=100;//pixels
/*
truecolor is 24 bits R(8)G(8)B(8) 255:0:0 (bright red)
32 bits is RGBA (alpha) a cool image with a transparent background, like a png round icon with wallpaper on a webpage
*/
$img=imageCreateTrueColor($w,$h);
//rgb 0-255 0=dark 255=total color or r,g, or b (like a dial for each) 66 dark red 255 chinese red
//same difference here
//$fC=new Array("66","89","104");
//$fCArr=explode(",","255,127,0");//take a string, and make an array with comma as the node seperator (explode it)
//$fontColor=imagecolorallocate($img,$fCArr[0],$fCArr[1],$fCarr[2]);
$fontColor=imagecolorallocate($img,255,127,0);
//same difference
//$fontColor=imagecolotallocate($img,$fCArr[0],$fCArr[1],$fCarr[2]);
/*
imagettftext
image bitmap
size of the text font
angle like italic in degrees? clockwise positive #(/) cc negative #(\)
x point to start text (left-right)
y point to start text (up-down)
font color in a imagecolorallocate with the bitmap and rgb in r,g,b (above)
font family and place the ttf font family is stored on the server so gd2 can read it to draw letters (KAIREE.TTF) file has to be where you say it is to reference and load into gd2 memory to draw
text to draw
*/
//$text="this should work!";
$len=8;
$chars="abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890";
$ranLen=strlen($chars);//how long is...
$text="";
//
for($a=0;$a<$len;$a++)
{
$pnt=rand(0,$ranLen);//random point to start
$ranChar=substr($chars,$pnt,1);//one char at that point
//echo $ranChar;
$text.=$ranChar;
}
//
$font="KAIREE.TTF";
$fontSize=24;
$x=80;//move 80 to the right from the left edge
$y=32+$fontSize;
imagettftext($img,$fontSize,0,$x,$y,$fontColor,$font,$text);
$filename="test.png";//save the bitmap we dynamically create ($img) to the hard drive on the server ($filename) as test.png
imagepng($img,$filename);
imagedestroy($img);//just clear up the server's memory from the scratch space used to temporarily create the image, like closing photoshop shen you've saved your doodle
?>
<html>
<body>
<h3>Random captcha creator</h3>
<h4><?php echo $text;?></h4>
<img src="test.png" />
</body>
</html>